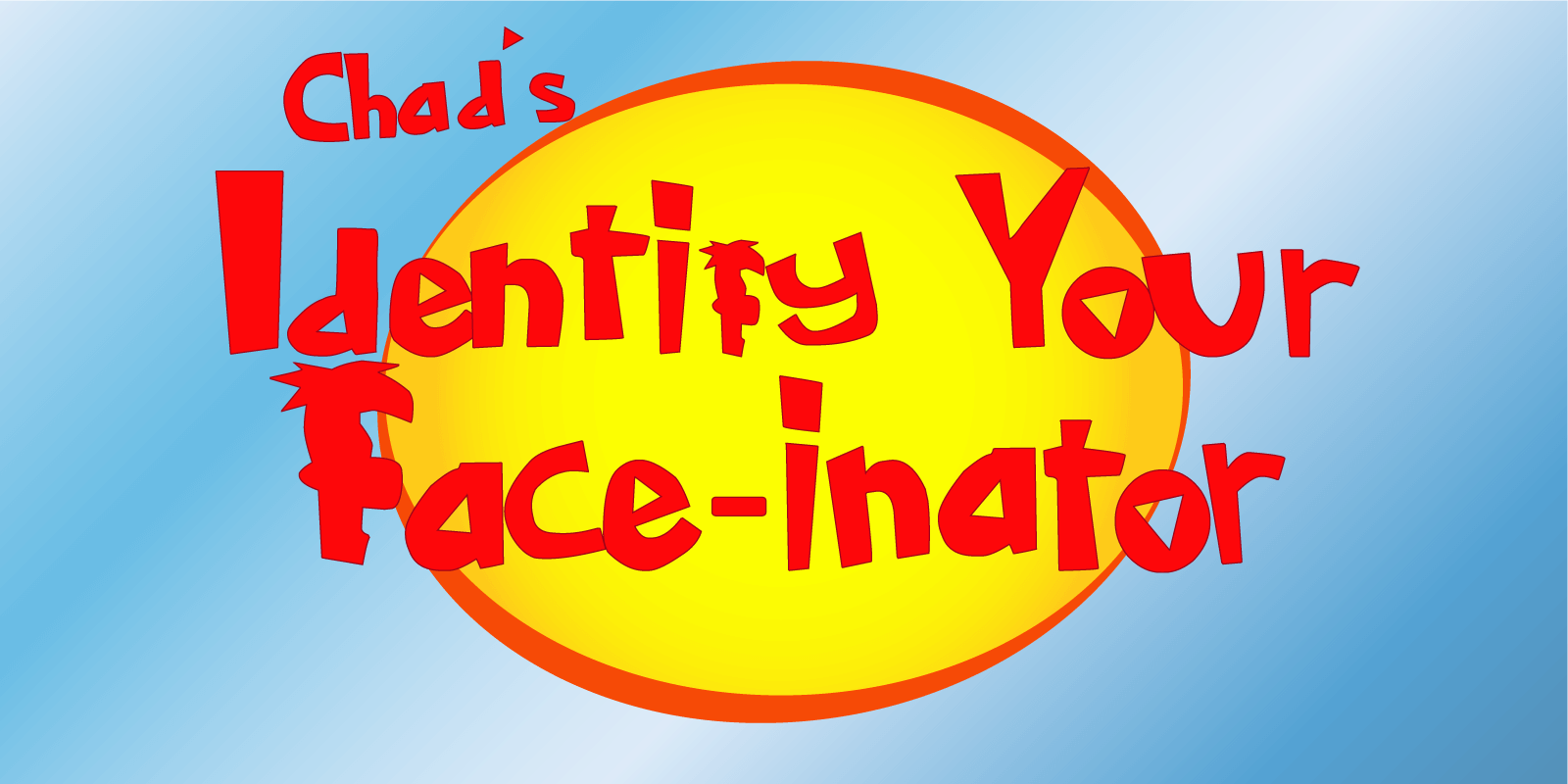
Chad’s Identify-Your-Face-inator
There are many solutions for face recognition. Azure and AWS both provide cloud-based solutions that can be consumed. However, there are other options that don’t require a cloud provider. One of these is OpenCV. In this blog post, we will explore using OpenCV to do some basic face recognition.
The steps for pretty much all face recognition is going to be the same.
- Train the system using known faces
- Run the face algorithm on unknown faces and see how it performs
Luckily doing these steps with OpenCV is pretty simple. For step 1, we must train over a known set of images. To do this, we need two objects from OpenCV:
face_finder=cv2.CascadeClassifier('haarcascade_frontalface_default.xml') face_recognizer=cv2.face.LBPHFaceRecognizer_create()
We will use the face_finder object to find faces in the image.
grayImage=cv2.cvtColor(image,cv2.COLOR_BGR2GRAY) imageFaces=face_finder.detectMultiScale(grayImage,scaleFactor=1.2,minNeighbors=5)
We will then add those faces to a faces array.
faces.append(grayImage[y:y+w,x:x+h])
After we have our faces, we can train the face recognizer.
face_recognizer.train(faces,np.array(labels_int))
Once we have a trained recognizer, it is ready to recognize faces. Doing so is pretty simple. We use the face recognizer’s predict method to find the face.
face_label=face_recognizer.predict(grayFace)
The result from the face_recognizer is an integer. We can use this integer to link back to the person associated with this face.
The code to pull this off with OpenCV wasn’t very complicated. You end up with 150 lines of Python code that can recognize the faces of people. My code is pretty unsophisticated; it assumes there is a directory of photos. Each person that can be identified will have a sub-directory under the main directory. Each of those sub-directories is full of images associated with that person.
I set up a people directory with my family and tried to use the system to predict (guess) who was in the photos.
Now a few things affected this. First, my Mac’s camera is not great; it’s a mediocre 720p camera. Photos that came from my laptop were prone to be problematic. Second, a family is an interesting challenge because members often resemble one another. For example, my son and I usually got registered as the other. To compensate for this, I ran a lot of pictures through the system to help train it. With enough photos, the predictions seemed to stabilize towards the correct answer.
This OpenCV solution surprised me with what you can achieve with just a little bit of code. I have a solution that can recognize each member of my family with only 150 lines of code. That is pretty impressive.
But I did run into a few issues. First, off you have to run a good number of images through the training algorithm before it starts making good recommendations. Second, people of the same family tend to be identified as the same person. My son and I still sometimes confuse the algorithm. Third, I think my solution would work a lot better with a better webcam.
Code
https://github.com/chadmichel/facinator
References
https://github.com/informramiz/opencv-face-recognition-python