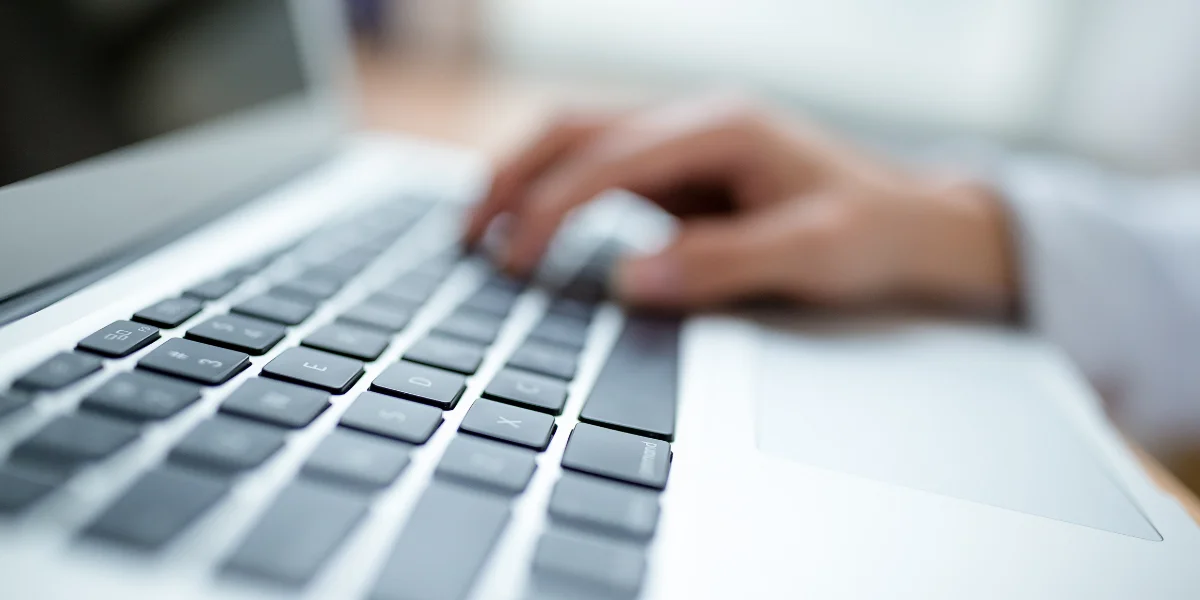
Creating and Running a Docker Container: A Step-by-Step Guide
Running a Docker container locally is a convenient method for packaging and deploying applications. In this blog post, we’ll walk through the step-by-step process of running a Docker container from an ASP.NET Core project directory. This guide covers installing Docker, creating a Docker image, running the container, and accessing the application endpoint. Let’s dive in!
Step 1: Installing and Running Docker
Before containerizing our ASP.NET Core project, ensure Docker is installed and running on your local machine. If not, follow the official Docker documentation to download and set it up.
Step 2: Creating a Docker Image
To containerize our ASP.NET Core solution, we need to build a Docker image. The image creation process involves specifying a build context, typically the folder containing the solution file (.sln). However, this approach can become cluttered when dealing with multiple clients or projects.
To address this, keeping each project’s Dockerfile within its folder is advisable. To build a Docker image when the Dockerfile is not in the parent folder, use the following command:
docker build -t <container-name> -f Dockerfile ..
The two dots (..
) at the end indicate to Docker to go up to the parent folder where the solution.sln
file is located. Replace <container-name>
with a suitable name for your container.
Practical Example
Assuming the following project structure:
To build a Docker image for client1
, navigate to the client1
folder and run:
docker build -t client1-image -f Dockerfile ..
This command, executed in the client1
folder, goes up to the parent folder where solution.sln
is located, and Docker builds the image accordingly.
Benefits of This Approach
- Clean Project Structure: Organize Dockerfiles within project folders, maintaining a clean and organized project structure.
- Separation of Concerns: Each project has its own Dockerfile, reducing the chances of mistakes and ensuring clarity.
- Ease of Collaboration: Minimize conflicts in Dockerfile management when collaborating with a team, as each project has its isolated Docker configuration.
Dockerfile Example
For clarity, let’s provide an example of a Dockerfile for client1. In this example, let’s assume serviceA and serviceB are both being used in client1:
In this Dockerfile:
- The first stage (build) is responsible for building the application.
- The second stage (publish) publishes the application.
- The final stage (final) uses the ASP.NET runtime image and copies the published files.
- Also, we’ve added the USER
nobody:nogroup
command before exposing the necessary port. This sets a non-root user for enhanced security, adhering to best practices and minimizing potential vulnerabilities.
Step 3: Running the Docker Container
Verify the successful creation of the Docker container by accessing its contents. Use the following command to enter the container and execute commands:
docker run -it --rm --entrypoint=/bin/bash <container-name>
Replace <container-name>
with the actual name or ID of the container.
Step 4: Modifying the Container’s Port
Docker containers may expose services on different ports by default. To change the port, use the following command:
docker run -it –rm -p 5000:80 –name
This maps port 5000 on the host machine to port 80 in the Docker container, allowing access to the container’s service through http://localhost:5000.