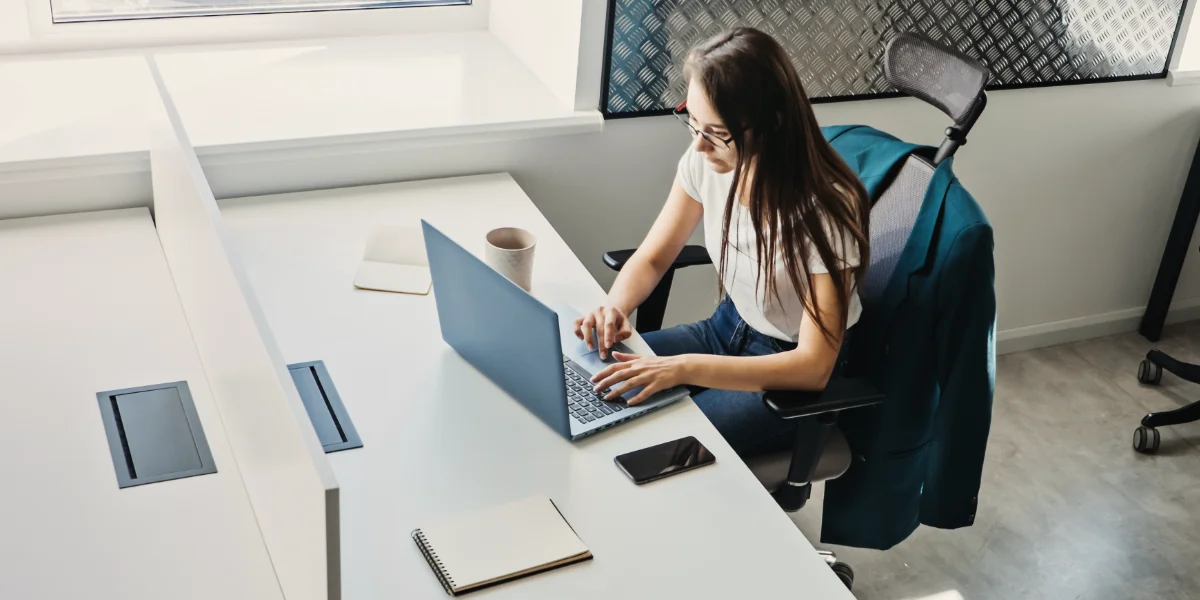
Using JSON Server for Efficient Development in Angular
When I am working on an Angular application, there are times I want to run the app without the backing API running as well. I want fast feedback on the changes I make to components and want my site to work by itself.
One way I have done this is to use a fake Angular service that acts like the real one. I use the fake service when developing locally. That works to achieve my goal, but it tends to be painful to maintain, or it can hide problems in my actual service.
Recently, I have found another way to keep my Angular application using its real services and still use mock data instead of calling my API. I have been using a tool called JSON Server to accomplish my goal of running my Angular application without my backend API running.
JSON Server gives me the power to set up specific, unchanging mock API responses. This allows me to build and test my Angular application in a repeatable, expected way. I can run my Angular app without modification, testing all its functionality exactly how it will run against the real REST API, but done in isolation from that REST API.
JSON Server is an especially useful tool for rapid iteration in frontend development. However, it requires some setup to work with Angular nicely.
Here is what I do to make it do what I need.
First, I added the JSON server npm package to my project as a dev dependency. This makes it usable for local development and CI builds but will not bloat the final application bundle.
npm install json-server --save-dev
I then create a “mocks” folder at the same level as my “src” folder.
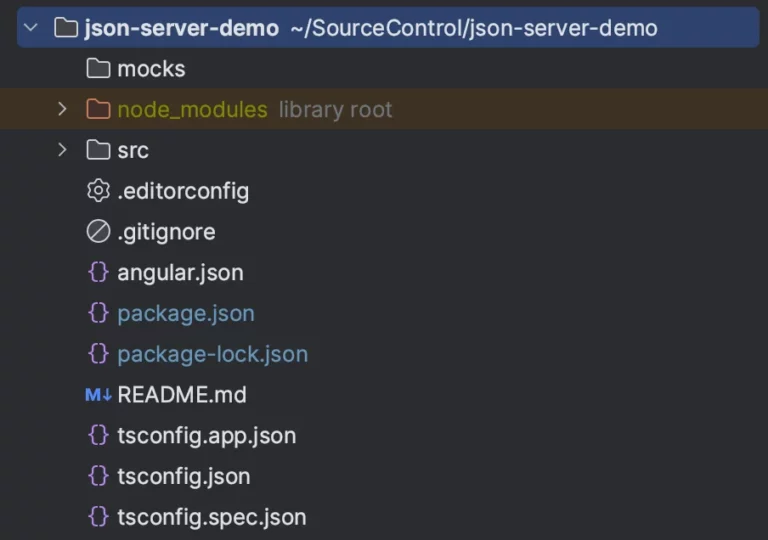
I add a routes.json file into the mocks folder.
I populate that file with the routes I want to have JSON Server watch for.
Next, I create a db.json file in the same folder so I can define the responses that I just used in the routes.json.
I also need to create a proxy.config.json file in the mocks folder that will tell Angular to redirect traffic to the JSON server instead of the normal API.
Lastly, I created a middleware.js file that will be used for even more customization and gives me a full JavaScript that I can do anything I need that the defaults do not account for.
This looks for all POST requests and changes them to GET requests. This allows me to prevent JSON Server from writing to the db.json file because I want those requests and responses to never change.
I additionally add a check for the special route that I call out instead of having my db.json contain 52 weeks’ worth of data. I generate that data programmatically using JavaScript and return that bypassing the db.json file.
My mocks folder now looks like this:
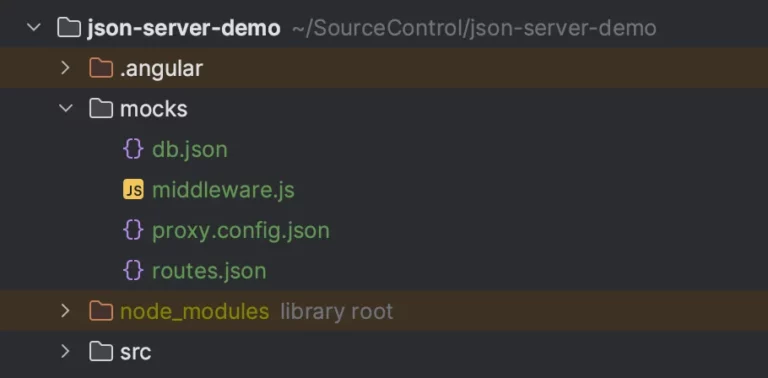
I need to do a little more work to get my Angular application to run at the same time as JSON Server and for it to proxy so that JSON Server intercepts the calls to the API correctly.
There is a serve section in my angular.json file (provided by angular cli as part of the project setup). I am going to make a new entry in this section named “mock” that I will use to point to my proxy.config.json file.
Now, I create a new entry in my package.json file under the scripts section to be able to run that mock configuration:
"start:mock": "ng serve --configuration mock"
So now, if I use this “start:mock” command, my Angular application will run with that proxy configuration, which allows me to keep my Angular project isolated from the JSON Server and running like it will in the real environment. I have connected the Angular app with the JSON Server.
I also want to make it easy to run JSON Server with the appropriate configuration, so I create another script in the package.json.
With these two commands, I have everything set up and working to make my Angular application think it’s calling the real API but instead have JSON Server returning responses.
Another benefit to this setup is that the db.json file can be used within the Angular tests to give them the same data without duplication.
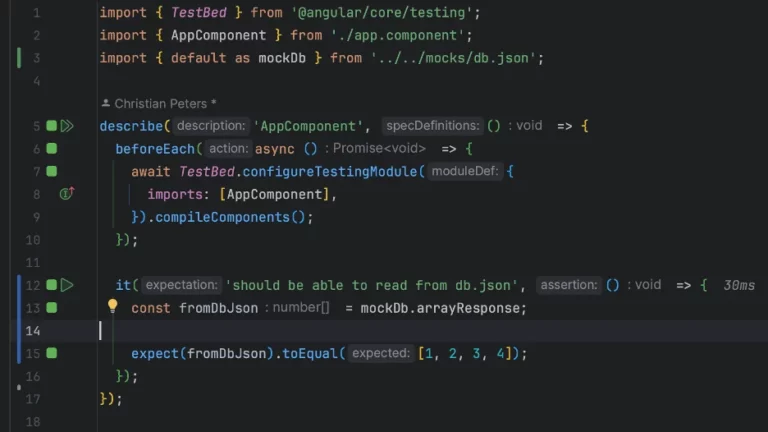
Conclusion
I have found that using JSON Server with Angular applications has simplified the development process, facilitated testing, and allowed for efficient frontend-backend interaction without the need for complex mocking. This tool is worth exploring for software engineers looking to enhance their development workflow.