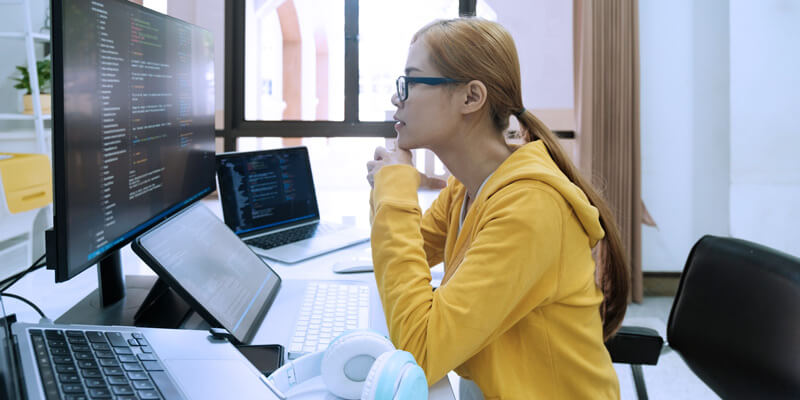
Setting Up Injection Tokens with Dynamic Values
Injection tokens are a popular way of providing dependencies in Angular. They are used to provide a value, such as a service instance or a configuration setting, to a component or other part of the application. Below is a simple example of setting up an injection token and using it.
Suppose you have a constant value that is needed in several components. You can define it as a static value and provide it to the components or services requiring it:
In the above example, the injection token provides its invariable value to other components or services. In the example below, I want to show an injection token of type BehaviorSubject
* where other components or services can change its value.
*BehaviorSubject
is an RxJS construct that maintains a value and broadcasts changes to that value to its subscribers. BehaviorSubjects are commonly used in Angular applications to share data between components.
In this example, we define an InjectionToken
called Default_Value
that is typed as a BehaviorSubject
. We also define a component called MyComponent
that injects this token. We want any components or services that use the injection token to be able to assign their desired default values to the string object.
The constructor of MyComponent
injects the Default_Value
token and sets the value of defaultValue
to the current value of the behavior subject by calling getValue()
.
Now, let’s say you want to provide an instance of this BehaviorSubject
with a default value of “Hello, world!” to the dependency injection system so that it can be injected into the MyComponent
. You can do this in your module as follows:
Now, when MyComponent
is created, it will receive the instance of the behavior subject with the default value of “Hello, world!” and set its defaultValue
property accordingly. If other components want to set a different default value in MyComponent
, they can do it easily thanks to InjectionToken
of type BehaviorSubject
.