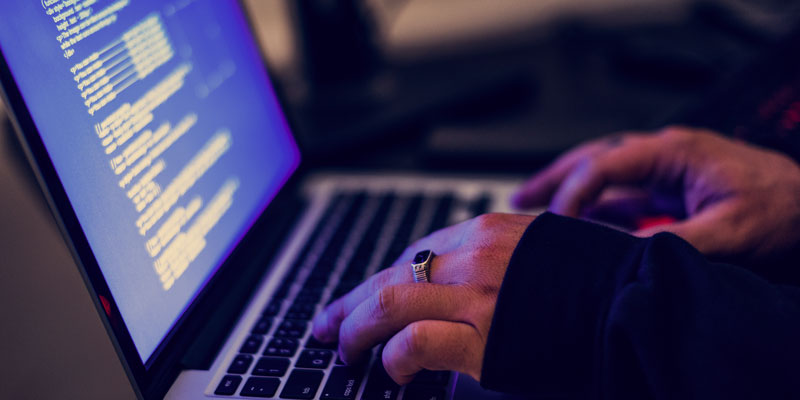
Quick Look – Angular Async / Await
These days, Async / Await is old hat for most C# developers. Using it in C# land makes async programming almost as readable as synchronous code.
What is the difference between synchronous and asynchronous code? Quite frankly, you can read synchronous code and asynchronous code, but it is kind of a mess. With asynchronous code, the flow through a program is a lot harder to follow. The C# world was mostly synchronous for a long time, but with .NET 4.5 asynchronous code was made more of a first-class citizen.
JavaScript has always been very asynchronous. Anytime you called out to another system in JavaScript, you were providing a callback function that would run when the other operation completed. This is how we wrote our AJAX calls 10 years ago.
Someone eventually realized that having callbacks all over the place was messy and complicated to read. They found a way to make asynchronous code easier to read by adding in the concept of promises. Promises are pretty good, but they still make reading async code an exercise in mental gymnastics. And promises don’t really solve the problem; you still cannot read your code from top to bottom. It is still async.
But some brilliant developer came up with the concept of async / await. With this concept, you only use a couple of keywords, but your code will behave as though it is synchronous code. This is a fantastic change. You get the benefits of async, with the readability of sync (or close to).
In the Angular code below, we have a function called “all” that returns a promise. This code is an excellent candidate to make async.
Making the “all” method async is super easy, just add the “async” keyword.
The calling code is pretty straightforward with promises. You just call the method “all” then pass a callback function into the “then” method. This is pretty standard JS callback type behavior, made slightly cleaner with promises.
Changing the calling code to use await is very simple. Again, just drop in a keyword. But now we get to see the power of “await”, we don’t have a callback. We actually get the results inline like synchronous code!
Async / await allows us to write more readable async code. Almost as good as the old synchronous code.