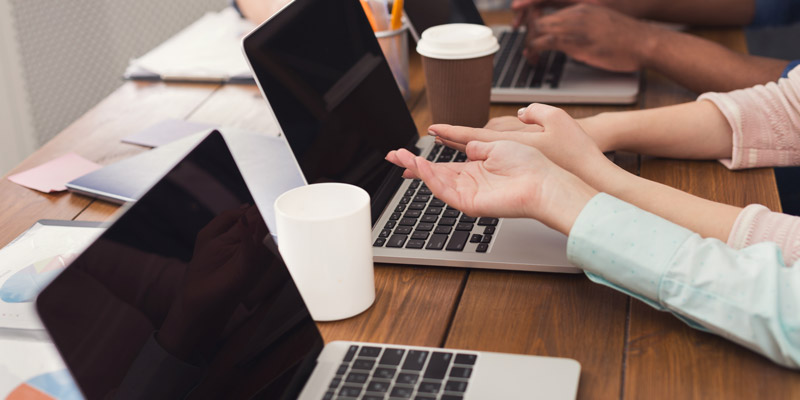
Quick Look – Angular Data Binding
In my previous post, we took a quick look at Angular’s routing. In this post, we are taking a quick look at Angular’s data binding.
One thing that’s pretty lame about our previous example is all the data is just backed into the page. With this blog post, we are going to pull static data from a service.
Creating a service is easy with the Angular CLI.
ng generate service Contacts
With a Contacts service, we can abstract away the call to a server backend. This will allow us to encapsulate all the server communication when retrieving contacts. If you are familiar with IDesign systems, this will feel right at home. We are basically writing a ContactsAccessor.
While this version of the Contacts service will just return static data, I will actually make a call to a .NET backend in a future post.
The Contacts service does depend upon another TypeScript file. We now have a Contacts DTO we need to create.
Now that we have a Contacts service with DTO, we need to consume that service from our Contact List component.
Now it’s time to consume the Angular service. We start by wiring up the DI through the constructor, then calling the method. In the code below, we want to call the ContactsService.All method. We get a reference to the Contacts service through constructor of our component. Then in the ngOnInit method we call the ContactsService.All method to get all the contacts from the service.
Finally, time for some data binding. We have data coming from a service to our component. How do we bind the data into a table? Pretty simple. We “for” (*ngFor) loop through the items in the array. We display each item using the {{}} syntax.
Tapping one of the items will navigate you to a Detail page where you can make changes. The ContactDetailComponent will need to call the Find method on the ContactsService. This will load the contact from its static list.
The view associated with the ContactDetailComponent will contain some more data binding. This time we will have two-way data binding. We will use the [[ngModel)] attribute on the input controls to bind those controls to properties on our component.
In this post, we have seen two types of data binding: the one-way data bind in the Contact List and the two-way data binding of the Contact Detail. Angular makes it really easy to bind your data to views.
Full source
https://github.com/chadmichel/AngularBlogPostSeries/tree/master/DataBinding