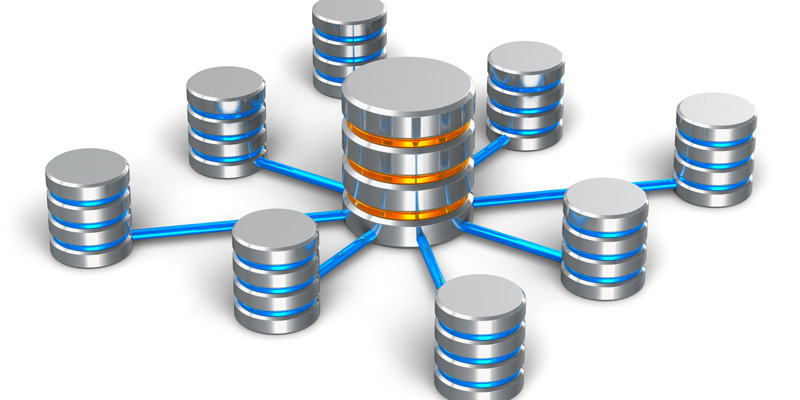
Database Change Control, Part 1
Source control is a foundational piece of any software development process. If you are not doing source control, you are doing it wrong. You will feel some pain someday, probably some bad pain.
Source control is useful for everything, not just your C# code but also your databases. Why should your database code be any different? Why would you not want everything to be the same?
But before jumping into database change control, I think you should ask yourself, “Should I even use a database for my solution?” I am a big fan of deferring your data storage technology for as long as you can. For more information see my blog post, Don’t Design the Database First.
If you have (and need) a database, you have database scripts of some sort. Not having those under some kind of change control is a big mistake. This blog post series will take us through a few options for storing database scripts in a .NET world.
So you can follow along, our base solution for these samples is at https://github.com/chadmichel/databasechangecontrol/tree/master/CodeFirst.
The example isn’t complex. We have a console application that parses CSV data and writes it to a single TimeLogItems database table. Don’t let the lack of complexity get in the way of the bigger point I’m trying to make: always make database script management part of your process.
For our first example, we are going to do something that I would almost never do for a large production system: let Entity Framework create the models.
Now if you are worried about the word “never”, you should notice that I said that for large projects. For smaller products and proofs of concept, I think letting Entity Framework create your database makes sense.
To let the automatic table / database creation take place, you basically have to do nothing. Just write the code, run the database creation, and viola!
Now in all reality, that isn’t going to be good enough. First off, you probably want some control over the database connection string. The easiest way is just setting the connection string name when defining your database context.
Database context
App.config
But what if you want to control how it creates the tables? That can be done using attributes you apply to your class or properties. You can add some simple attributes to control things such as the max length of a string field. You can also use an attribute to define the primary key on a table.
You can get a complete list of annotations from MSDN.
In this post, we hit on some of the basics of using Entity Framework Code-First to manage our database creation / update. Code-First is a super-effective way to develop you proof-of-concept database, although I think there are better options for managing database updates for projects that have multiple versions. We will cover some of those options in future posts.