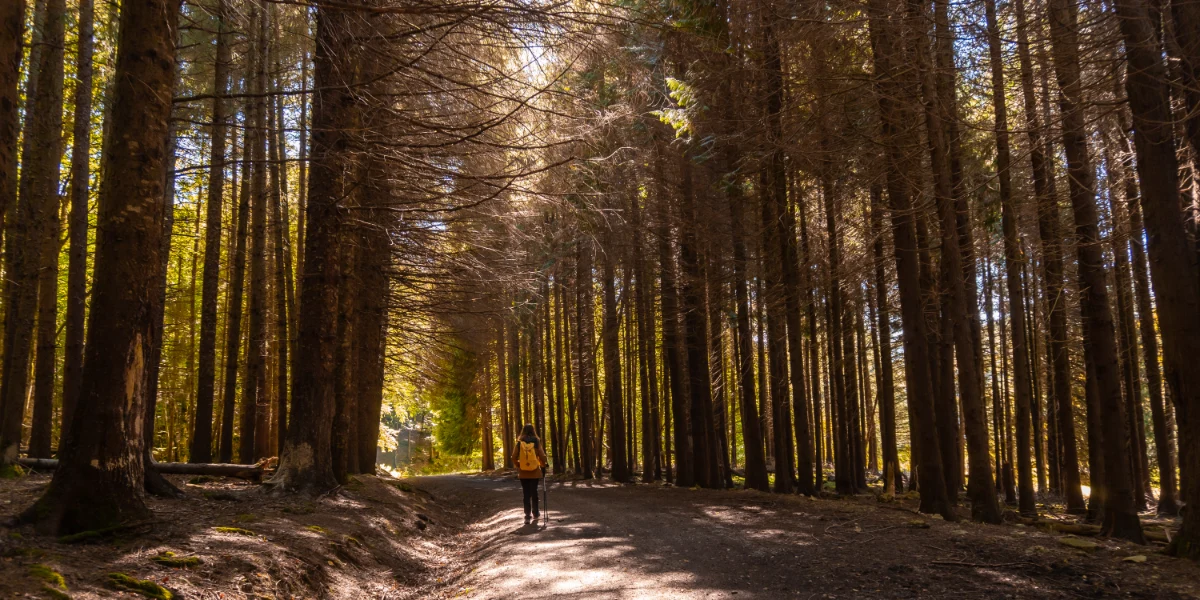
Angular Adventures: A Journey to Building an Angular Application – Part 4
Mastering Data Management: Arrays and Loops in TypeScript
The final core pieces we will explore in the last post of this series are arrays and loops. As a beginner, I found that arrays and loops are essential tools in TypeScript, tying together many of the foundational concepts we’ve learned so far.
Let’s start with arrays. Arrays let us store and manage multiple values, whether they’re numbers, strings, or objects.
Instead of creating separate variables for each value, we can use arrays to organize data efficiently. You can think of arrays as a list, or a collection of items, where in my example our collection is different types of sports. You can access each value in the array by calling its position. For the above example: Volleyball = index 0, Football = index 1, Basketball = index 2, and Soccer = index 3. You always start your index with 0 instead of 1.
If you want to replace a specific sport in the above array, like replacing “Football” with “Tennis”, you can access the position of “Football” (which is index 1) and assign a new value like this:
This example demonstrates how easy it is to not only access the items in the array but make changes to the array as well.
Not only are arrays beneficial for collecting items, but they can also hold multiple values of the same type (e.g., numbers, strings), making them incredibly valuable in our code. As a beginner, I found it helpful to visualize arrays as containers that keep things organized. We showed an array of strings above; below is an example of an array of numbers:
Tying in Loops
Loops (like for
or while
loops) allow us to repeat actions, like accessing or replacing each element in an array. For example, in our array of scores, we can use a loop to calculate the total or find the highest score, making it easier to handle large amounts of data.
A loop will continue to iterate until a specified condition, like a stopping condition, is met. Here are a few examples of how different loops can be used to work with arrays:
- While Loop: The
while
loop is used when you need to loop until a condition is met or the condition returns false:
- Do While Loop: This form uses the keyword
do
, followed up by a code block, followed by the keywordwhile
and its condition. A do-while loop guarantees at least one iteration because the condition is evaluated after the code block runs. The loop continues as long as the condition remains true:
- For Loop: Used when you know how many iterations/blocks of code you need to run. This method of loops uses three statements separated by semicolons:
- For Each Loop: This is the most modern form of a loop in C#. The
forEach
loop goes through each item in a list or array and runs a block of code for every item until there are no more left. It’s written by using thefor each
keyword, followed by the item type, a name for the item, the word in, and the list or array to loop through:
- For…Of Loop: Used to iterate over the values in an array, the for…of retrieves each item directly, making it useful when you need the values rather than the indexes.
- For…In Loop: The for…in loop iterates over the indexes (or keys) of an array or object. It’s useful when you need to access both the index and the value at that position.
Arrays and loops work seamlessly with the other concepts we’ve explored in TypeScript:
- Assignment Statements: We use them to initialize arrays and assign values during loops.
- Objects and Classes: Arrays can store objects, allowing us to manage complex data structures. For example, an array of objects could represent a list of users, where each user is an instance of a class.
These tools are essential for managing collections of data and executing repetitive tasks, allowing you to write more efficient and organized code. As a beginner, I appreciate how TypeScript adds value by ensuring that our arrays, loops, and data types are used correctly. The type system guarantees that we’re working with the right kind of data, reducing errors and making our code easier to understand and maintain – every coworker’s dream!
Together, arrays, loops, and these other concepts form the building blocks for creating more complex, efficient, and error-free applications in Angular. Stay tuned for the next part of the series, where we’ll explore even deeper into additional features and best practices on how to build an Angular application!