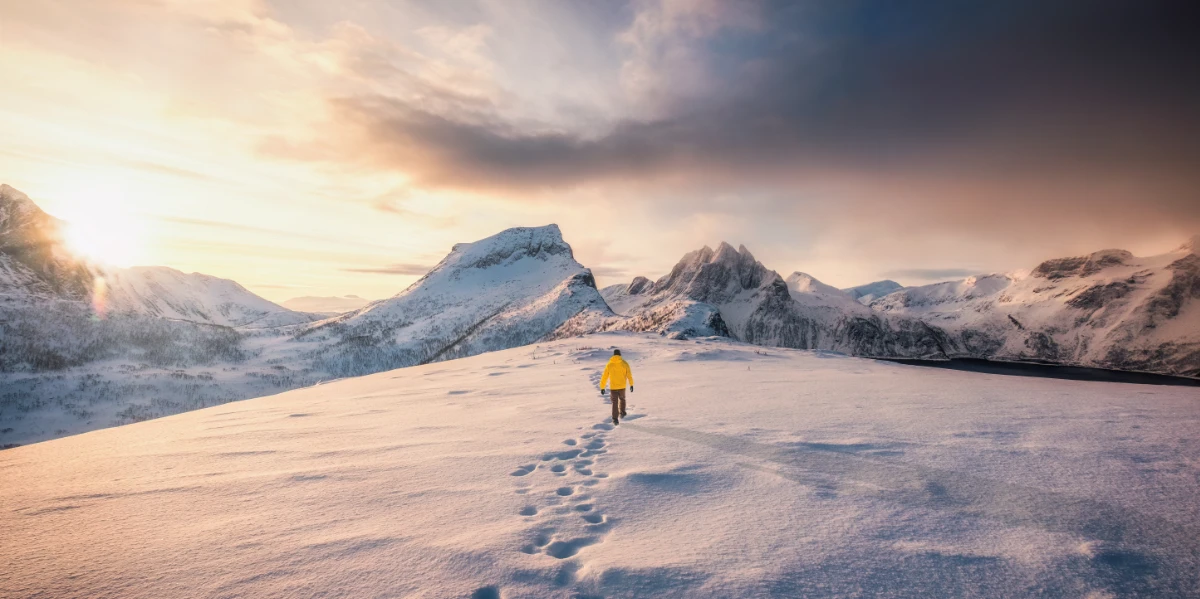
Angular Adventures: A Journey to Building an Angular Application – Part 3
Understanding Classes and Objects in Angular
So far in our Angular Adventures, we’ve covered two main concepts that will help us along with our Angular development journey: TypeScript, and why we use it, and Assignment Statements, and the meaning and purpose of each. In this post, we’ll dive into two more key fundamentals: Classes and Objects.
What are Classes?
As someone with a background in HTML, when I heard the word “class” in TypeScript, I thought I would easily grasp it, but I quickly realized it’s quite different. In HTML, the class attribute is used to apply CSS styles to an element or reference it in JavaScript. You can assign multiple class names to an element, making it easy to apply consistent styles and behaviors across your code.
HTML Example:
The class=header”
applies the class “header” to the div
element. You can then reference this class in your CSS file to apply specific styles to any element with the class “header.”
In Angular, classes are part of TypeScript, which follows object-oriented programming principles. These classes are used to define components, services, and models, making them essential for organizing and managing the logic of an Angular application.
Angular Example:
The class NotificationSender
stores information about the message to be sent and the recipient. It also includes a method send()
that defines the action of sending the message.
In Angular, classes help you define the behavior and structure of components or services, allowing you to create more organized and reusable code. When naming your classes, while not required, it’s considered good practice to start with an uppercase first letter. This is also known as Pascal Case, which makes your code more readable and consistent.
What are Objects?
Simply put, an object is a collection of related data and functionality. Objects are created from classes, which act as blueprints for creating them. Objects often represent real-world entities, in our case a dog, and they combine both the properties that describe an object and the methods that define the action the object takes.
TypeScript Constructors
A constructor is a special method within a class that is automatically invoked when a new instance of the class is created, allowing the object’s properties to be initialized. In other words, it’s like a setup function that runs when you create a new object, giving it its initial values.
Let’s add a constructor to our NotificationSender
class. We can set the values for the message and recipient when a new notification sender object is created:
Now, when we create a new NotificationSender
object, message, recipient, and the constructor will automatically assign values to the object’s properties, making it more efficient to initialize objects with specific data:
Inheritance and Polymorphism
Two important concepts that come into play when working with classes in Angular are inheritance and polymorphism.
Inheritance allows a class to inherit properties and methods from another class, enabling code reusability. I’ve created a subclass called EmailSender
that inherits properties from NotificationSender
but adds specific behavior related to sending emails:
Polymorphism enables objects to be treated as instances of their parent class, even when the objects belong to different subclasses, allowing flexibility when methods are being used. For instance, you could have various types of notification senders, like EmailSender
and SMSSender
, both sharing a method called send()
, but each with its own unique implementation.
In the example below, we define the SMSSender
class that also extends NotificationSender
while overriding the send()
method:
Both SMSSender
and EmailSender
inherit from NotificationSender
, but they implement the send()
method in different ways. Polymorphism allows us to handle different types of notification senders under the NotificationSender
type, giving us the flexibility to call the same method on different objects while letting each object perform its unique implementation. Take a look at the following example:
This demonstrates the power of polymorphism in object-oriented programming.
By combining all of these key concepts: classes, objects, inheritance, polymorphism, and constructors, Angular offers a powerful way to organize and manage your code. This makes it easy to maintain your system with reusable code.