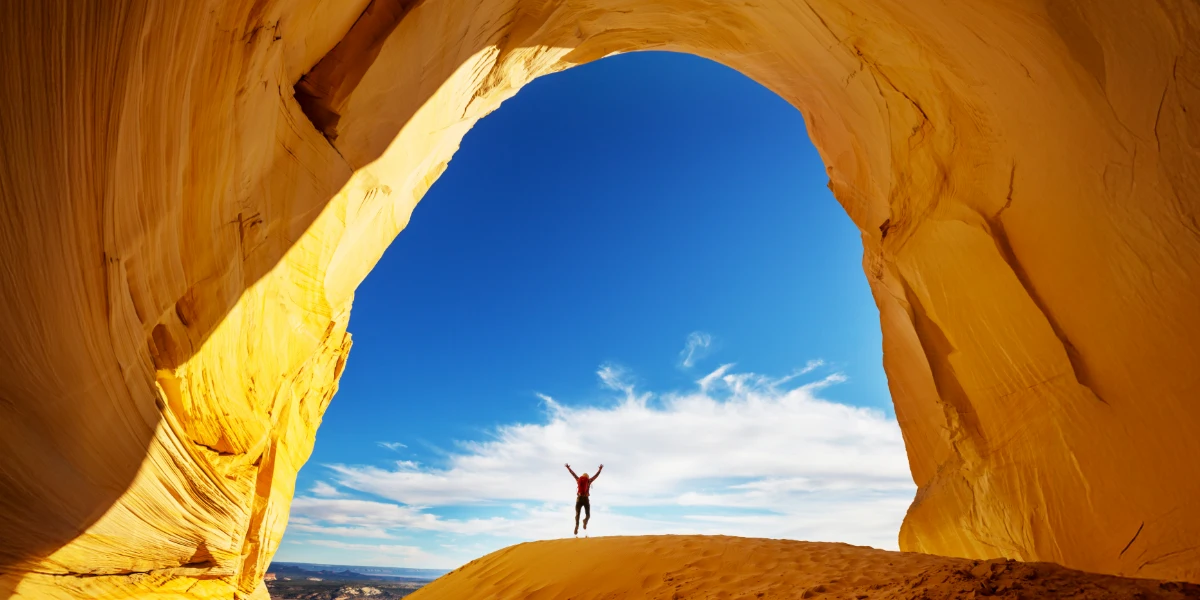
Angular Adventures: A Journey to Building an Angular Application – Part 2
The Backbone of Your Angular App: Assignment Statements
In my last post, I explored the world of Typescript and the benefits of using it for creating Angular Applications, whether you’re a beginner or an experienced developer. In this post, we are diving into an essential feature of Angular: assignment statements.
What are Assignment Statements?
Simply put, an assignment statement is used to assign a value to a variable. You will see this concept everywhere throughout your Angular app, and you need to successfully identify how to use it in the right context.
Declaring Variables
It’s important that we understand how to use the keywords var
, let
, and const
in their proper states and understand how they all behave differently.
First, let’s start with var
. var
can be used in local variables to initialize a statement that can be re-declared or updated, but it is not recommended for use in modern Typescript due to the variable not being explicitly defined. Using var
could cause maintainability issues down the road. If you want to be able to change a variable while still being confined within a block of code, let
will be your keyword of choice. You can reassign while using let
, but redeclaration is not allowed. If you don’t want others to be able to reassign variables after the value is set, you will want to use const
. This declares the variable as a constant, unchangeable keyword.
While I’m still learning TypeScript and consider myself a beginner, it was difficult to understand which keyword to use until I really understood their behaviors. If you misuse a keyword, an error might not specifically be thrown, potentially leaving you with sloppy, incorrect code. If you have a strict linter (a tool that analyzes your code for potential errors or bugs) installed, it will suggest changing the keywords to the appropriate option.
The Importance of Assignment Statements
Assignment statements play a huge role in Angular applications, specifically storing values in variables and making sure your code is dynamic and reusable. Here are a few key points as to why assignment statements matter to us:
- Store Data – they let us store data to use at a later time. You can assign a user’s input to a variable and use it throughout your code.
- Simplifying Operations – instead of repeating calculations or operations, you can store the result in a variable once and use it whenever needed.
- Memory Management – assignment statements help allocate and deallocate memory efficiently by keeping track of what data is being used and when it’s no longer needed.
Assignment statements provide structure, and who doesn’t love a well-organized system? They enable the dynamic flow of data throughout a program, making your code more efficient, adaptable, and easier to maintain.
Types of Assignments
In addition to the basic =
operator that I provided earlier, TypeScript offers multiple advanced operators to use throughout your code:
+=: Adds the value on the right to the value on the left and reassigns it.
-=: Subtracts the value on the right from the left and reassigns it.
*=: Multiplies and reassigns.
/=: Divides and reassigns.
These concepts may appear simple, but they are the backbone of any Angular application. Assignment statements enable you to store, manage, and control your data while keeping your app dynamic and responsive. As we continue our Angular Adventures, remember that mastering these simple, foundational features will make development much easier in the long run, and understanding when to use the right keyword is crucial for progressing in your development career.