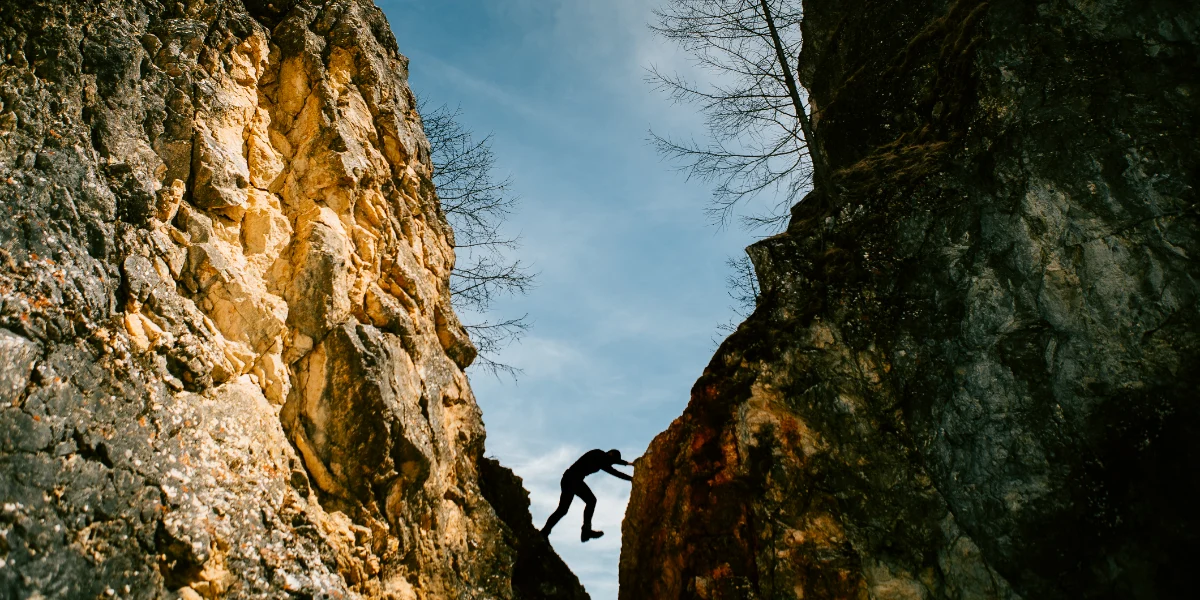
Angular Adventures: A Journey to Building an Angular Application – Part 1
Welcome to Angular Adventures: A Journey to Building an Angular Application. In this blog series, I will discuss the ins and outs of what it takes to write an Angular application, exploring key concepts and tools that are essential to mastering Angular. I’ll start with TypeScript and why we use it at Don’t Panic Labs. Then I’ll dive into assignment statements and how to know the difference between them all and cover fundamentals like classes and objects. This blog series will wrap up with arrays and loops, a crucial piece to handling data in our application.
Whether you’re a beginner or an experienced developer looking to sharpen your Angular skills, this series will take you on a journey through the core building blocks of Angular.
TypeScript and Why You Should Use It
I recently started learning TypeScript and quickly realized how beginner-friendly it is. One of the main reasons I say this is the error detection built into TypeScript. It made it easier for me to learn as I went along, spotting errors and mistakes and essentially “coaching” me along the way. Error detection is one of the largest challenges developers face during a project, especially as the project grows in size and complexity. TypeScript is a superset of JavaScript that adds static typing, bringing more reliability and efficiency to your development process and as a beginner, this is crucial!
Let’s explore the key reasons why adopting TypeScript can be beneficial for your next project, whether you are a beginner or an experienced developer.
Error Reduction and Detection
TypeScript enhances JavaScript by incorporating static typing, which catches errors early in the development cycle. Since TypeScript is compiled down to JavaScript, it can catch common errors such as incorrect property names, improper function usage, and other type mismatches before the code even runs, all while significantly reducing runtime errors. I found this incredibly helpful as a beginner developer. It made development smoother and helped me learn where I was making the mistakes right in front of me. Fewer bugs mean a smoother user experience, and as a front-end developer that is my main priority.
Let’s look at some error detection examples.
JavaScript Example (Without Types):
In the above code, there is no type checking, so passing a number instead of a string won’t result in an error.
TypeScript Example:
In the TypeScript example, the function greet
expects a string
type for the name
parameter. If you try to pass a number, TypeScript will throw an error during development, helping to prevent potential issues.
Maintainability
One of the standout features of TypeScript is its emphasis on maintainability. With strong IDE support, developers benefit from advanced tooling, autocompletion, and refactoring capabilities. The static typing system of TypeScript makes it easier to manage large codebases by clearly defining what each piece of code is supposed to do. At Don’t Panic Labs, multiple developers often access the same codebase throughout their tenure. As a beginner, it’s important to understand that keeping your code organized and well-maintained can lead to success down the road – whether it’s your own success or that of a coworker who needs to access the code you previously wrote.
Integration with Popular Frameworks
TypeScript’s versatility is evident in its seamless integration with multiple frameworks and libraries. At companies like Don’t Panic Labs, where development is done across various frameworks such as Angular and React, TypeScript proves to be a valuable tool. Angular, in particular, is built with TypeScript, which makes the integration process exceptionally smooth. This level of compatibility allows developers to leverage TypeScript’s features while working within their preferred frameworks, ensuring a streamlined development process.
Understanding TypeScript’s Type System
TypeScript introduces a robust type system that adds an extra layer of security and reliability to JavaScript. This system includes type inference, which means that TypeScript automatically understands what data types should be associated with variables based on their initial values. This feature minimizes the need for manual type declarations and reduces the likelihood of errors. In JavaScript, developers often struggle to understand the types of data being passed around due to its loosely typed nature, but TypeScript solves this by letting us developers specify data types and making the code more predictable, which ultimately leads to avoiding confusion in your code and making sure your code behaves as it should.
JavaScript Example:
TypeScript Example:
In TypeScript, we define an ‘interface’ to specify the shape of the user
object. This ensures that user
adheres to the expected structure, making the code more predictable and easier to debug.
Improved Refactoring Capabilities
Refactoring is a crucial part of maintaining and updating codebases. With TypeScript, refactoring becomes much more manageable due to the static type system. The language provides powerful tools to safely rename variables, extract methods, and move code around without breaking functionality. This capability is essential for teams that frequently update their codebases, ensuring that changes can be made confidently and quickly without introducing new bugs.
In summary, TypeScript is a powerful addition to the JavaScript ecosystem for both beginners and experienced developers. By adding static typing, it reduces errors, enhances maintainability, and provides seamless integration with popular frameworks. Its type system and refactoring capabilities make it an excellent choice for developers to write cleaner, more efficient, and more maintainable code. So, if you are looking for a way to improve your development process – especially as a beginner – it’s time to consider incorporating TypeScript into your development toolkit.