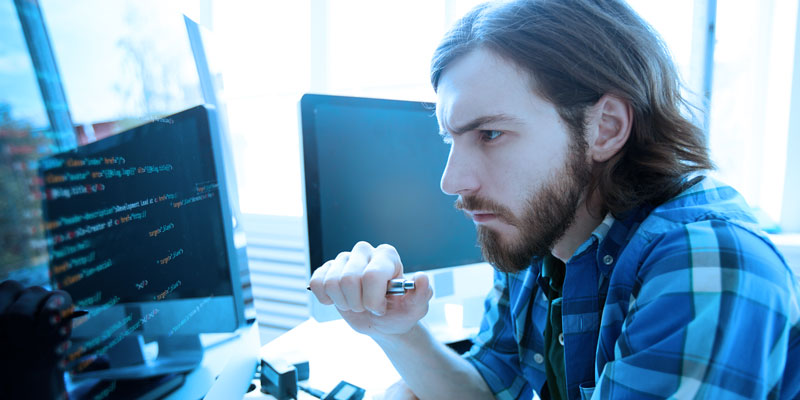
Quickly Finding Sentiment Using Azure Text Analytics
Text analysis can potentially be very powerful. The ability to judge text as positive or negative is very interesting. This would obviously be a very powerful tool for analyzing things such as blog comments or product review comments (“Are people giving us positive or negative feedback on our product?”).
So lucky for us, Text Analytics is pretty easy to work within Azure. We can get something up and running with three basic steps.
Create an Azure Service for Text Analytics.
Write some very simple code. This code just needs to create TextAnalyticsClient. Then call AnalyzeSentiment. Yep, it’s that simple.
Then we can look at the results. Azure gives us Positive, Negative, and Neutral results.
Results
Positive: 0.55
Negative: 0.23
Neutral: 0.22
Now, what about using this to look at some political articles. I took the top five CNN articles about Trump and about Biden by searching for “cnn trump” or “cnn biden” on August 10th using Google News.
What can we learn from this?
First off, there isn’t enough data for this to be relevant. We would need to run a lot more articles through our system to get anything relevant. But the one thing we maybe could glean from that is there is a lot of negative political articles out there. Only one of the 10 was positive.
Azure Text Analytics is pretty amazing, and it’s amazingly simple to interact with considering the power it provides. Whether you’re classifying information based on the vocabulary used or gathering customer sentiment, Text Analytics is a welcome tool to have in your toolbox.